In Laravel, view components offer an elegant way to structure your user interfaces into smaller, reusable code fragments. Achieving a delicate balance between reusability and customizability can be challenging at times. Reusability might take you only so far, leading to inevitable code duplication. In this tutorial, we'll explore a more flexible approach to crafting Blade components.
Consider the following example:
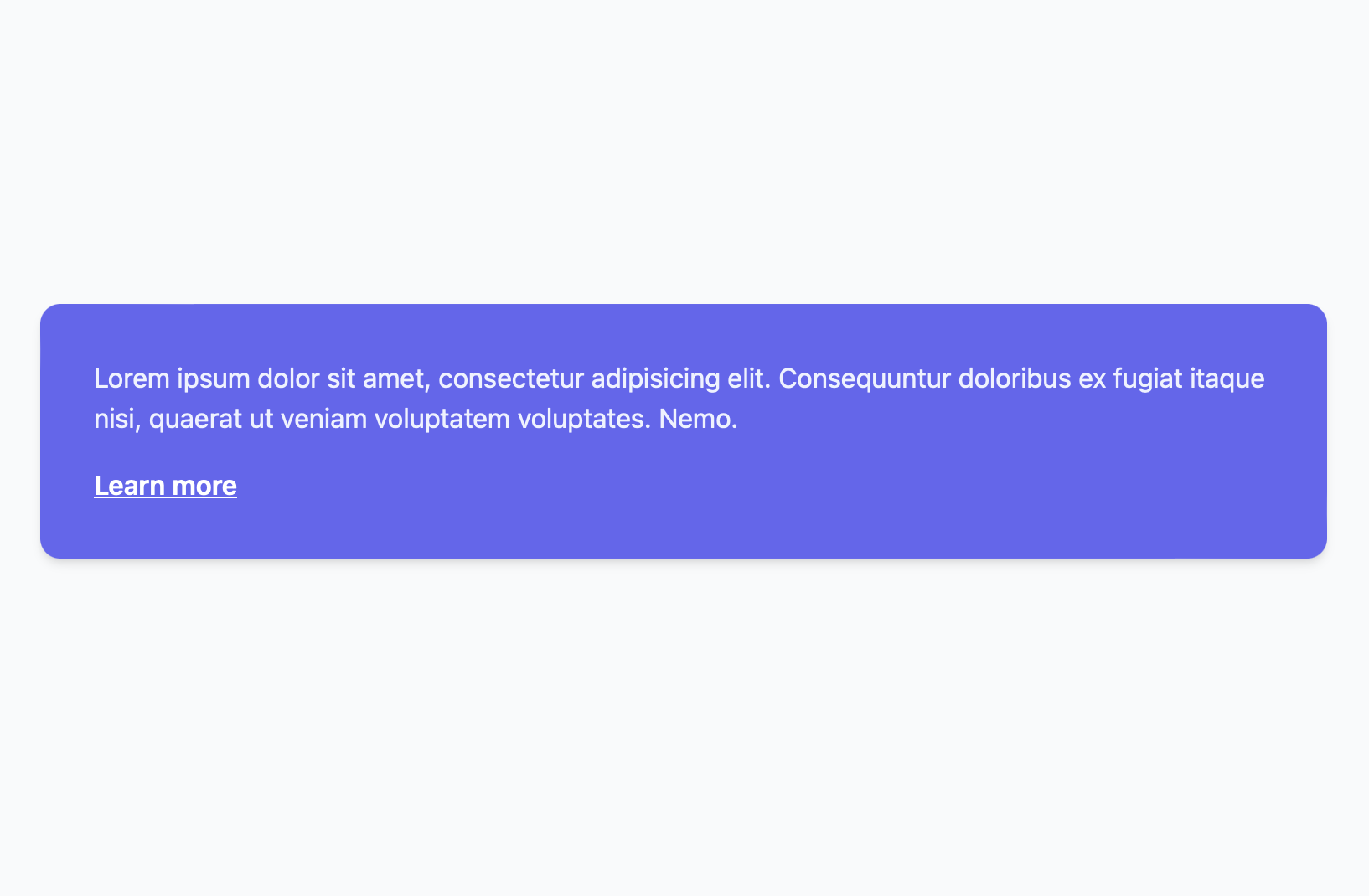
Here's the code for this component, which we'll refer to as message-box:
Of course, this isn't the only way to create this component, but it helps illustrate the problem we're addressing.
As you can see, this component is relatively straightforward to use:
You can customize the link (URL and label) and the message. However, what if you want to change the background color? You have multiple options.
One option is to introduce a new property for changing colors based on its value:
There's nothing wrong with this approach, except that you're introducing a new property to the component. You might need to do the same for other customizations, like changing the link's target or other attributes:
One possible solution
What if you could set attributes individually for each section of your component? For instance:
As of now, Laravel Blade doesn't support this feature. When you implement a Blade component, all the attributes you define are available in the component view via the $attributes variable, but you can only set one set of attributes for the component. You can read more about Component Attributes in Blade in the official documentation.
Let's explore a better solution.
The $attributes variable is an instance of Illuminate\View\ComponentAttributeBag::class and is also Macroable. This means we can extend its functionality through a macro.
We want to introduce the ability to extract different attribute groups for each section of our component, like link:, msg:, and box:. Here's how it could work:
The collect method is a new method we're introducing in the ComponentAttributeBag::class to extract all the attributes with the given prefix and repackage those attributes in a new attribute bag without the prefix. This allows you to send attributes dynamically to each section of our component using the right prefix. The implementation of the component would look like this:
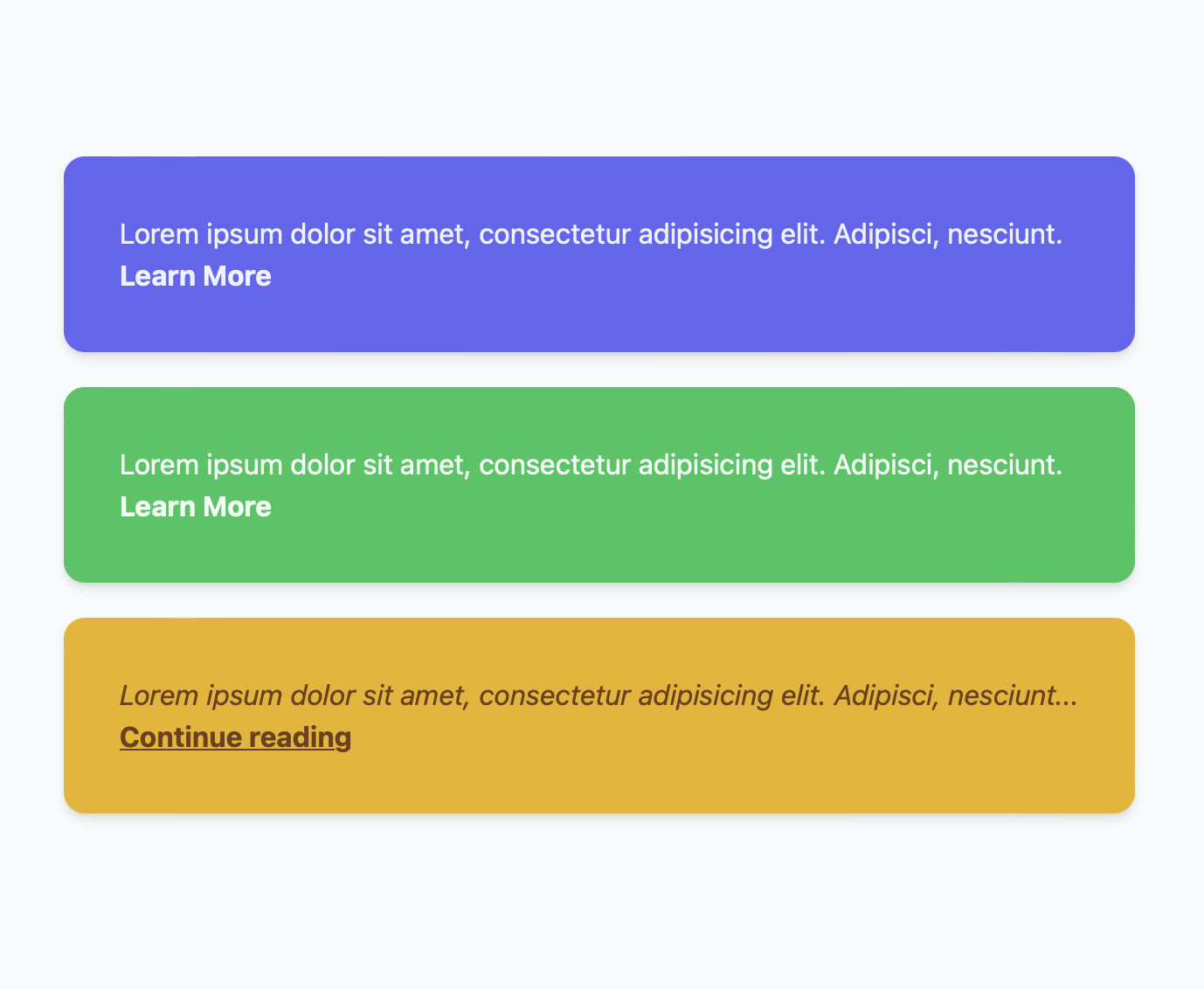
To make this possible, we're introducing a macro:
You can add this macro through a service provider's register method. You can learn more about how to Register a Service Provider in the Laravel documentation.
Conclusion
In this tutorial, we've explored a practical solution to enhance the flexibility of Laravel Blade components. By introducing the ability to set attributes individually for different sections of a component, we empower developers to fine-tune customizations without introducing unnecessary complexity. This approach ensures that your Blade components remain both reusable and adaptable to various scenarios. As you apply these principles to your projects, you'll discover a newfound level of control and efficiency in crafting user interfaces. Laravel Blade's versatility, coupled with these insights, opens up new possibilities for creating dynamic and highly customizable web applications.